Write a function in R
A function to count the number of digits
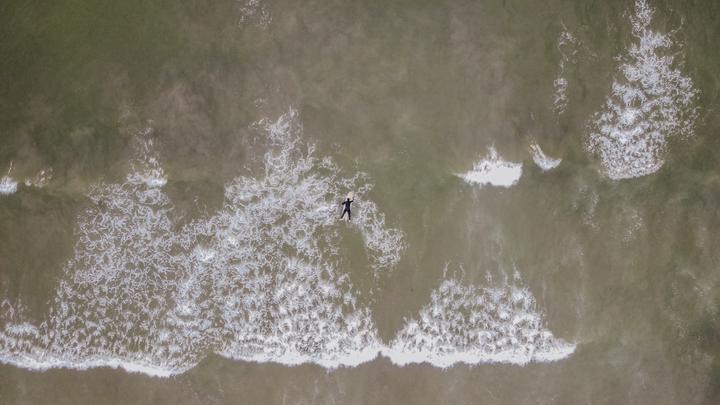
Welcome
The Programming with Data course introduces R programming to the Master of Professional Accounting students who have almost zero background in programming. After almost 5 hours of training on R programming, we tried the following Challenging Practice. The good news is that almost all students got it right.
Challenging Practice
I copy the practice question as follows:
Define a function called digits(n)
which returns the number of digits of a given integer number. For simplicity, we assume n
is zero or positive integer, ie, n >= 0
.
- if you call digits(251), it should return 3
- if you call digits(5), it should return 1
- if you call digits(0), it should return 1
For practice, you are required to use if
conditions and while
loops when necessary. You should use integer division %/%
in the while
loop to count the number of digits. You are not allowed to use functions such as nchar()
and floor()
.
And here is the suggested solution:
- return 1 if the input number is zero
- else do a while loop to count the number of digits. We will use the integer division for counting.
digits <- function(n) {
count <- 0
if (n == 0) {
return(1)
} else {
while (n / 10 >= 0.1) {
count <- count + 1
n <- n %/% 10
}
return(count)
}
}
digits(0)
## [1] 1
digits(5)
## [1] 1
digits(251)
## [1] 3
A more general function
If we don’t restrict the input numbers as non-negative integers, we can revise the function as follows. The only change is to make negative numbers as positive and get the integer of a floating number.
digits <- function(n) {
count <- 0
n <- abs(as.integer(n))
if (n == 0) {
return(1)
} else {
while (n / 10 >= 0.1) {
count <- count + 1
n <- n %/% 10
}
return(count)
}
}
digits(-15.23)
## [1] 2
digits(-1350.35)
## [1] 4
digits(251.53)
## [1] 3
Of course the nchar()
can do it directly.
digits <- function(n) {
n <- as.character(abs(as.integer(n)))
return(nchar(n))
}
digits(-3800.23)
## [1] 4
digits(-135078.3545)
## [1] 6
digits(259.534)
## [1] 3
Done
To reinforce your understanding of loops in R, you may read and practise this datacamp post.
Happy Coding! And get ready for our next Pop Quiz 😄.